In the rapidly evolving landscape of web development, choosing the right JavaScript framework is crucial for building efficient, maintainable, and user-friendly applications. With a multitude of options available, developers often find themselves in a dilemma about which framework to choose. In this post, we'll take an in-depth look at six popular JavaScript frameworks: React, Solid, Vue, Svelte, Angular, and Qwik. We'll explore their key features, pros, and cons to help you make an informed decision on which is right for you.
We’ll also include some code showing what a simple select component might look like in each framework, a few stats about the built project, and thoughts while building out the component. All code is available on github.com. Let's get into it.
React
React is developed and maintained by Facebook and has become a cornerstone of modern web development. It's a component-based library that focuses on building user interfaces and allows developers to create reusable UI components.
Building out a simple Select component is straightforward, though that could be because React is our preferred framework and the one we use the most. React is sometimes criticized because you have to explicitly handle setting the value and handling the change events, where some frameworks like Vue add some syntactic sugar to make setting this up easier. A project consisting of only this component had a build size of 143kB which is not terrible, but hardly great compared to some of the newer frameworks.
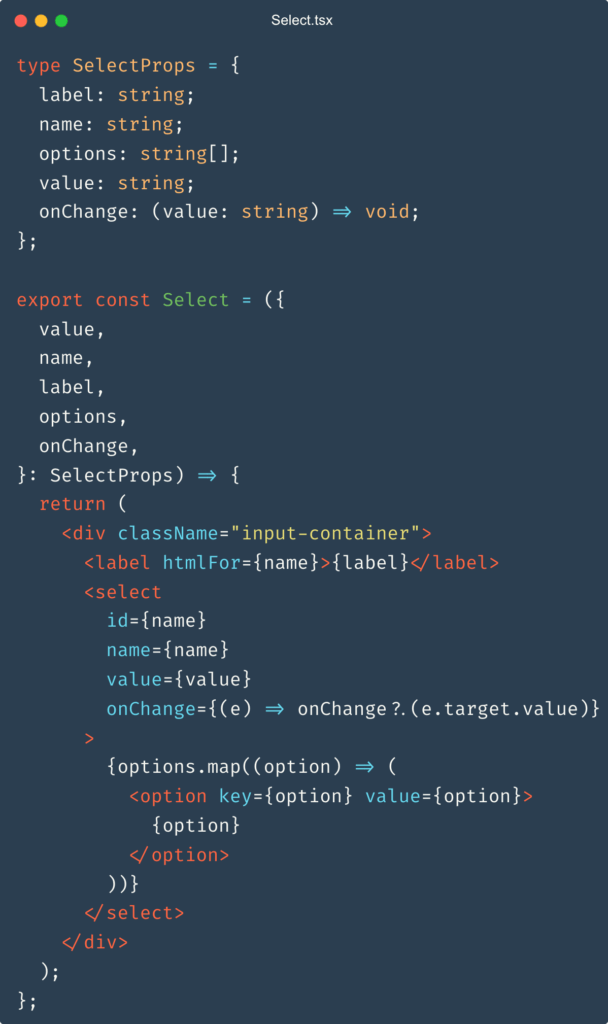
Pros:
- Virtual DOM: React's Virtual DOM efficiently updates only the necessary parts of the actual DOM, leading to better performance.
- Large Community: React has a massive community, resulting in extensive documentation, tutorials, and third-party packages.
- Component Reusability: React's component-centric approach promotes code reusability and maintainability.
- Strong Ecosystem: The ecosystem includes tools like Jotai, Zustand, and Redux for state management and Next.js for server-side rendering.
Cons:
- Learning Curve: The component-based paradigm and JSX syntax might have a steeper learning curve for newcomers. That being said once you learn JSX it can be used in other frameworks like Solid, Qwik, Astro with only minor differences.
- Performance Optimization: Understanding when and how to use “useMemo” and “useCallback” are essential to getting the best performance but are also easy to mess up.
- Boilerplate: Complex applications may require additional libraries, potentially leading to more boilerplate code.
Solid
Solid is a relatively newer framework that aims to provide a highly efficient reactivity system. It focuses on minimalism and optimal performance by reducing unnecessary re-renders.
One thing you might notice when looking at this Solid component is how similar it is to React’s implementation! The only differences are the <For/> component to loop over the options. Technically you can actually write the loop exactly like React’s, but for best performance Solid recommends using the <For/> helper to iterate. One thing to be careful of in Solid though is that when you destructure props like we are here, you could run into issues where you make a reactive prop non-reactive. It's not an issue here but it is an issue that is easy to make if you are coming from React.
The size of this project when built is only 14kB, which is tiny, making Solid a great choice if you need a tiny JS footprint and excellent performance, especially if you already know React.
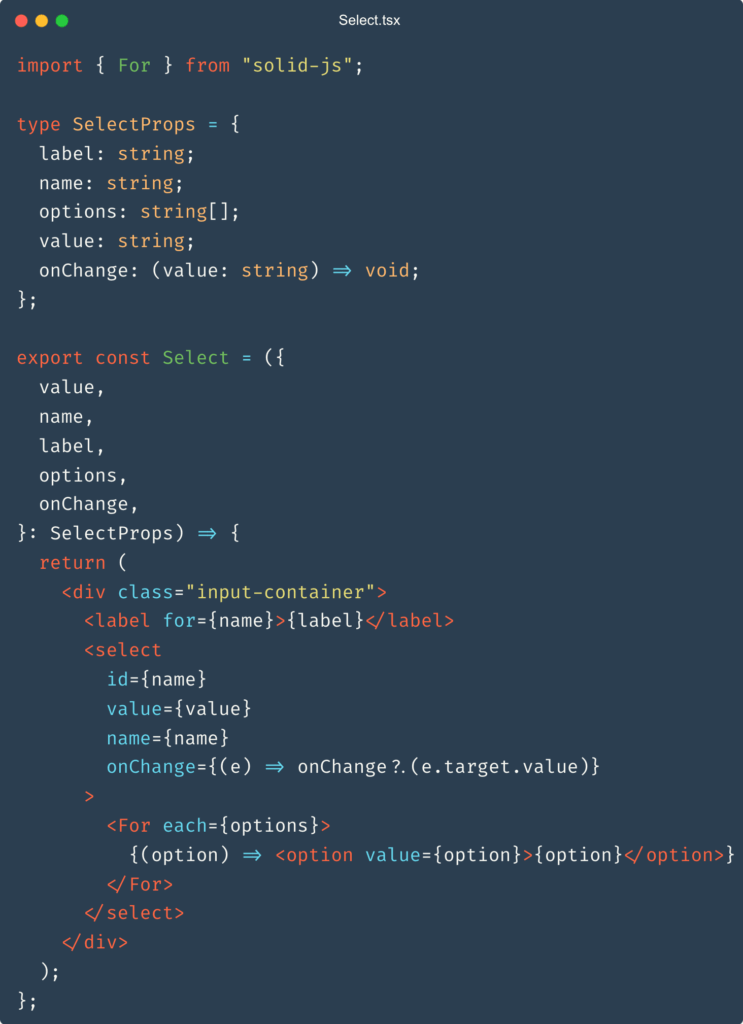
Pros:
- Reactive System: Solid's fine-grained reactivity system ensures efficient updates without unnecessary re-renders.
- Small Bundle Size: Solid's runtime is lightweight, resulting in smaller bundle sizes and faster load times.
- JavaScript Syntax: Solid's syntax is similar to Reacts, making it accessible to developers already familiar with that.
Cons:
- Smaller Community: Compared to older frameworks like React and Vue, Solid has a smaller community and fewer third-party packages.
- Limited Ecosystem: While Solid's core is robust, it may lack some of the extensive tools and libraries available in other frameworks.
Vue
Vue.js has gained popularity for its simplicity and ease of integration. It offers a flexible and progressive framework for building user interfaces. Recently it has released a new API called the Composition API, which can help organize your code and make your code easier to reason about. Supporting two APIs has muddied the water when it comes to finding guides/references online.
Vue diverges from React quite a bit with its default template syntax, though it is possible to use Vue with JSX. For the most part Vue wants you to feel like you're just writing HTML, with a few extra directives to extend it. Vue is a bit more magic than React and can get more complicated when writing highly reusable components, but the more complex components can potentially lead to easier implementation when using the components.
The build size of this project came out to be 51kB which is firmly middle of the pack, but definitely not bad at all.
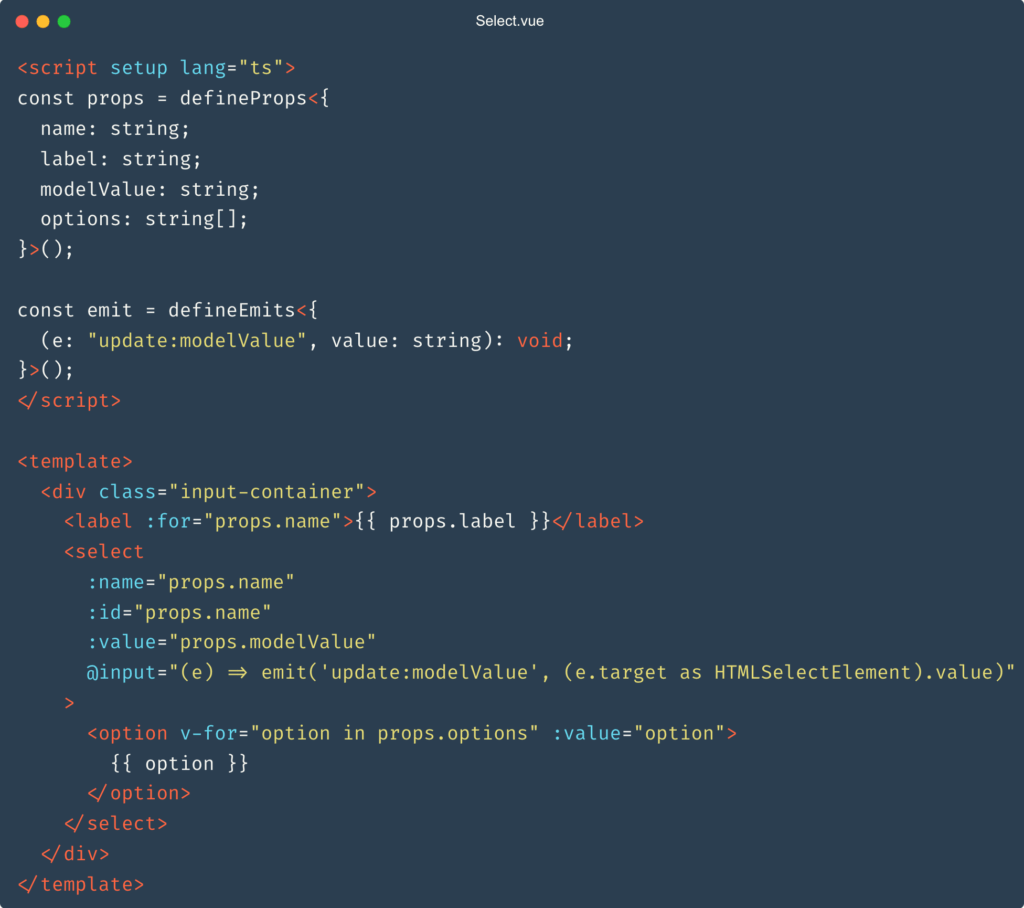
Pros:
- Approachable Syntax: Vue's templating syntax is easy to understand for both newcomers and experienced developers.
- Versatile: Vue can be used for building small components or full-fledged single-page applications.
- Comprehensive Documentation: Vue boasts clear and comprehensive documentation, aiding developers of all levels.
- Great Supporting Packages: Vue maintains some great support packages for some common things that most apps need. For routing, most people are going to reach for vue-router. For state management, most will be using Pinia, which are both excellent.
Cons:
- Scaling Challenges: While Vue is great for smaller projects, it might face scalability issues in larger applications compared to other frameworks like React, though one might argue the Composition API helps with this.
- API Confusion: There are a lot of ways to write Vue. There is the Options API, the Composition API, and the Composition API using the setup script. If that all sounds confusing, well it is! This is mostly an issue with learning the language, and discovering what works best for you, but can definitely be confusing.
- Smaller Ecosystem: Vue's ecosystem, though growing, might have fewer options when compared to React's ecosystem.
Svelte
Svelte is unique in that it shifts a significant portion of its work from runtime to compile-time, resulting in highly optimized applications. Svelte, while new, has been battle tested in large applications like the New York Times. Svelte can be run as an SPA, though for SSR there is Svelte Kit.
Working with Svelte is very easy, and a lot of thought has been put into DX. The templating engine is essentially just HTML with some directives for control flow.
This project’s build size is a paltry 8kB. This is because Svelte isn’t really a framework, it’s a compiler. That’s why some people call it the disappearing framework.
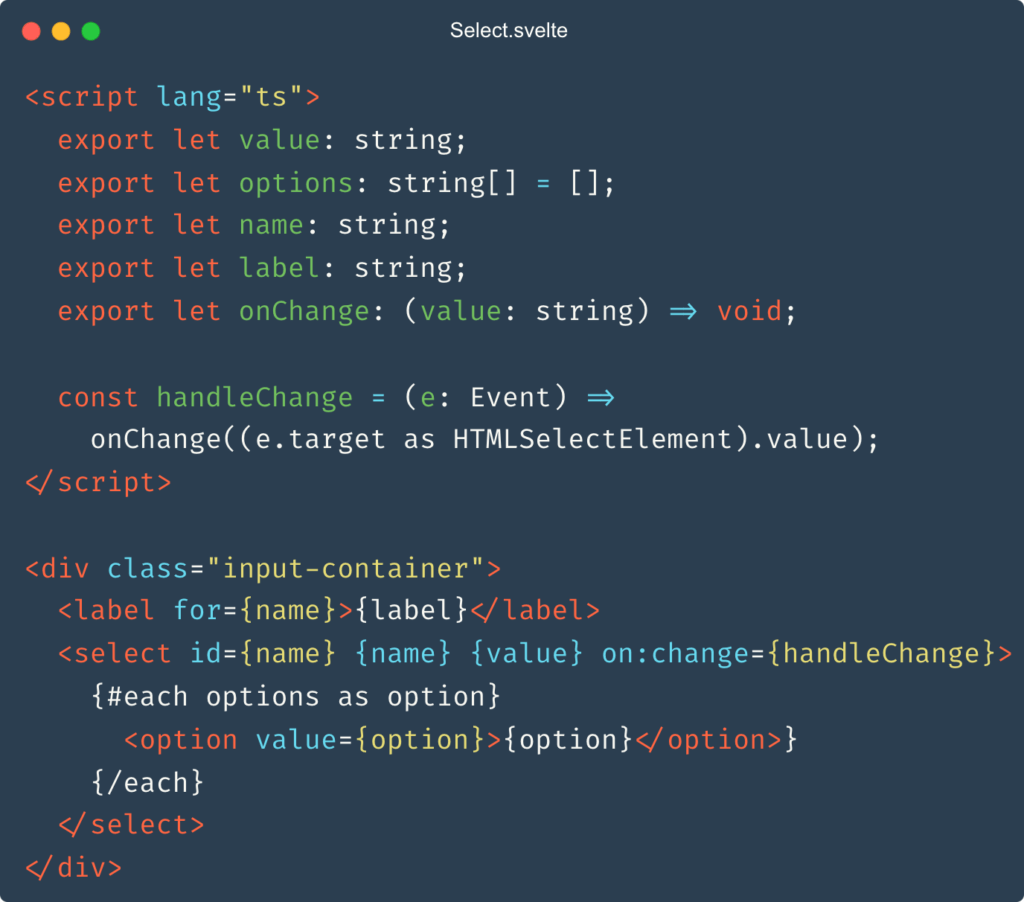
Pros:
- Zero-runtime Approach: Svelte compiles components into efficient JavaScript at build time, resulting in faster runtime performance.
- Declarative Syntax: Svelte's declarative syntax makes it easy to learn and understand.
- Automatic Optimizations: Svelte's compiler optimizes code automatically, reducing the need for developers to manually optimize.
Cons:
- Smaller Community: While Svelte's community is growing, it might not be as extensive as some older frameworks.
- Learning Curve: Developers accustomed to other frameworks might need time to adjust to Svelte's paradigm.
- Typescript Support: Because it has its templating system, the Svelte team also has to support its own LSP (Language Server Protocol) for TS support, and it doesn’t always work as well as JSX implementations.
Angular
Angular, developed and maintained by Google, is a full-fledged framework that offers a comprehensive set of tools for building dynamic web applications. Angular has a long history, and while it is one of the oldest, it still has a fairly large base of support, especially amongst bigger enterprise companies.
Of all the Select components, this one is the most complex in my opinion. Angular has a much different paradigm from any of the other modern frameworks. Angular still holds firm to the separation of concerns and thus you will typically have 3 files per component; HTML, JS, and CSS.
The build size of this Angular project clocked in at a hefty 179kB. That is a pretty large starting point, and the more modern frameworks have definitely made big strides in reducing bundle sizes. This is probably not a big deal for most web apps, but if you are targeting low mobile devices this could be an issue to consider.
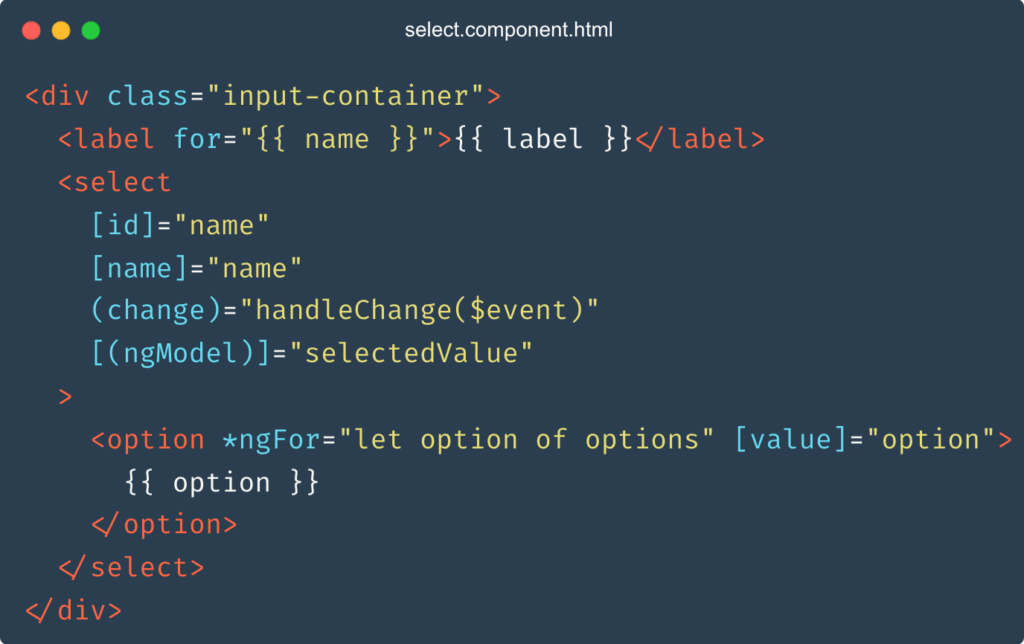
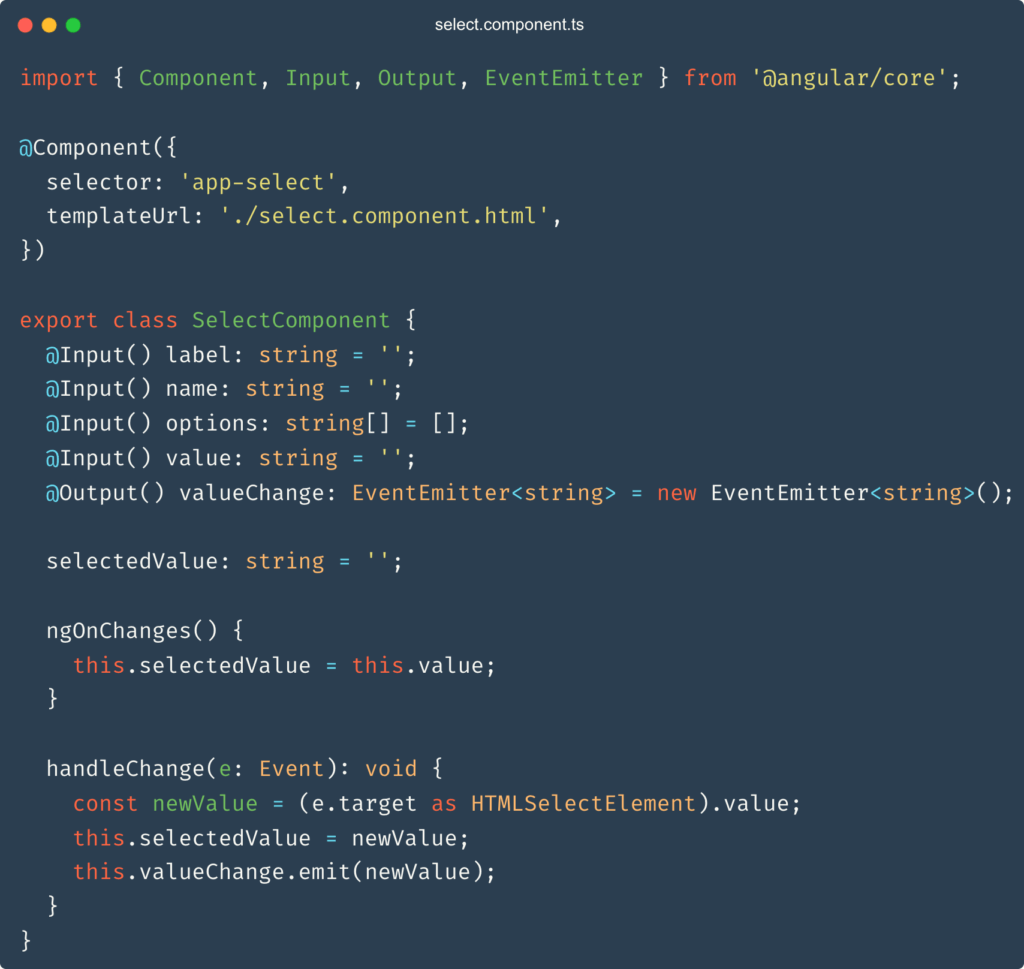
Pros:
- Complete Solution: Angular provides everything needed for building complex applications, including routing, state management, and more.
- Strong Typing: Angular is built with TypeScript, which offers strong typing and enhanced code quality.
- CLI Tooling: Angular CLI streamlines project setup, testing, and deployment processes.
Cons:
- Complexity: Angular's extensive features and concepts can lead to a steep learning curve, especially for beginners.
- Heavier Bundle: Angular applications might have larger bundle sizes compared to some other frameworks.
Qwik
Qwik is the new kid on the block and is probably not production ready for anything mission critical, but it is a very promising framework. What makes Qwik so exciting is that it ships only tiny amounts of JS to the client and the rest of the needed JS is lazy loaded.
The first thing you’ll notice about the below Select component is how similar it is to React and Solid. This makes getting up to speed easier, though learning all the new paradigms of how Qwik functions can take a while. For instance, any JS you put in the body of your component will never execute on the client. Instead, it will run on the server, and then resume on the client. This resumability means that your props all need to be serializable, or passed as a QRL.
Getting a build size for Qwik doesn’t make a ton of sense because Qwik doesn’t function as a SPA and requires that you have a JS server to host the app. In general, on first load, you can expect around 6kB or so of JS. It will never load JS that isn’t needed which is a huge shift in how most frameworks handle JS. Qwik is definitely a framework to keep an eye on.
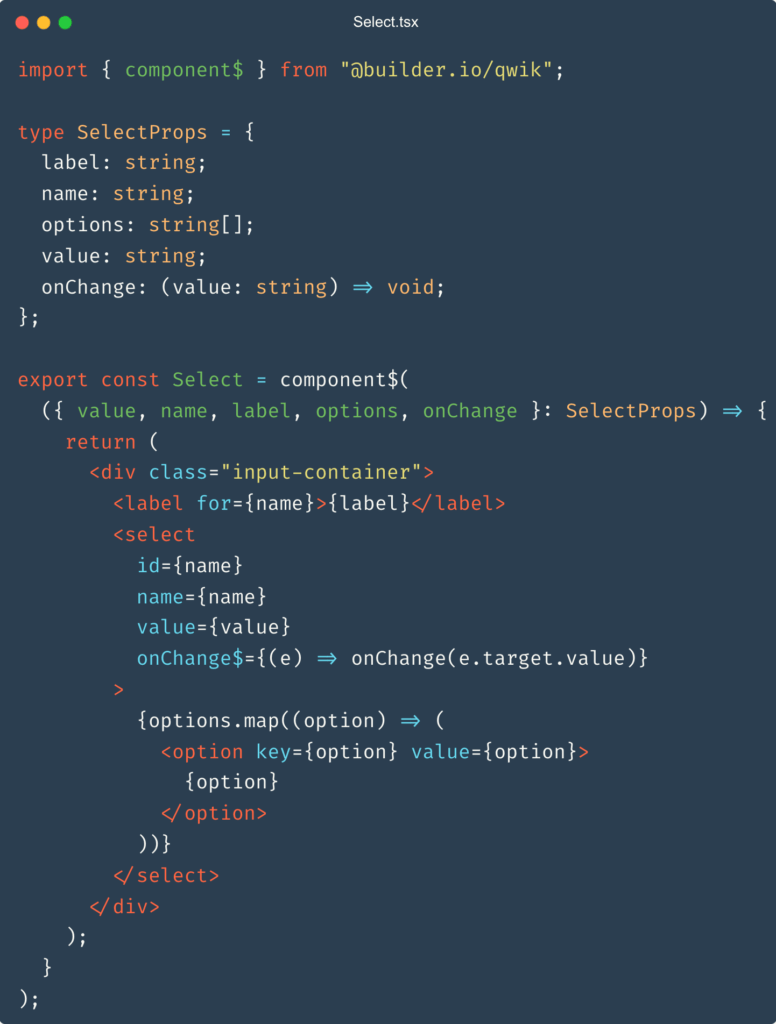
Pros:
- Server-Side Rendering (SSR): Qwik is designed for optimal server-side rendering, leading to improved initial load times.
- Component-Centric: Qwik follows a component-centric model, similar to other popular frameworks like React and also uses JSX.
- Emphasis on Performance: Qwik places strong emphasis on rendering performance and optimization.
Cons:
- Experimental Nature: As of now, Qwik is still in its early stages and might lack the stability and ecosystem of more mature frameworks.
- Limited Adoption: Due to its experimental status, Qwik might not be the best choice for large-scale production applications.
Conclusion
React, Solid, Vue, Svelte, Angular, and Qwik all offer unique features and trade-offs. So how do we pick the framework that is going to bring the most value now and in the long term for our project and org?
Evaluate these frameworks based on your project's needs to make an informed decision that aligns with your development goals. Project requirements, developer familiarity, performance considerations, and community support should all come into play when thinking through the options. Bundle size, SEO optimization, and the skill sets of your existing dev team are also worth thinking through.
With all these things to consider, how can you make sure you make the right choice? Well, that’s where we come in. At DeveloperTown, we’ve helped hundreds of clients make the right technology choices over the last 10 years. From picking the right tool sets to mentoring your existing development team, we’re here to help make sure your next project is a wild success.